The Azure AD Connector (AADC) syncs user objects between local ADs and the Azure AD / O365. In most situations, the user sync uses the GUID (Global Unique Identifiers) values as a matching attribute that connects the local and the cloud account. Just because the GUID is globally unique and it (usually) does not change over the object’s lifetime. In hybrid scenarios, it’s often good to know how to convert a GUID into a Base64 encoded string. Here’s why:
You may know GUID values in string representations like this: “123e4567-e89b-12d3-a456-426655440000”. But in fact, it’s a 128-bit binary value. Local AD has no problem storing this as a true binary. But Azure AD loves strings in attribute values. So we have to get used to converting this kind of GUID values into Base64 encoded strings. Because this is what Base64 is – a method to encode pure binary data into a simple ASCII string without any special characters.
So if you want to deal with attributes like objectGUID, ImmutableID, cloudSourceAnchor or sourceAnchor in PowerShell scripts, it is good to know how to convert these values into GUIDs or into Base64 encoded strings.
Convert a GUID string into a Base64 encoded string
We take this GUID string as an arbitrary example: 3ab39606-c642-489b-84b6-58c038d3ef39.
Its Base64 representation is this: BpazOkLGm0iEtljAONPvOQ==
To convert the GUID into the Base64 encoded string, use this PowerShell one-liner:
$guid = "3ab39606-c642-489b-84b6-58c038d3ef39"
$base64 = [system.convert]::ToBase64String(([GUID]$guid).ToByteArray())
$base64
Convert a Base64 encoded string into a GUID
We again take our example from the last paragraph. Only this time the other way round. To convert the Base64 encoded string into the GUID, use this PowerShell one-liner:
$base64 = "BpazOkLGm0iEtljAONPvOQ=="
$guid = [GUID]([system.convert]::FromBase64String($base64))
$guid
Dealing with GUIDs
As you might have noticed, the script examples use the [GUID] typecast to convert a GUID string (like “3ab39606-c642-489b-84b6-58c038d3ef39”) into a variable of type GUID. Which is, in fact, another thing than the string. For example, if you read a local AD object GUID with PowerShell, the property type returned by the API is pure GUID:
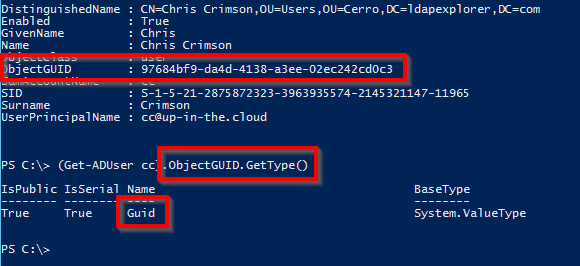
Please be aware that there might be some situations in which you need to have the string representation of a GUID. OR you might need the pure byte array of a GUID. This is the way to achieve this:
$guid.ToString()
$guid.ToByteArray()
If, on the other hand, you have a string and want to form a GUID from it, use this command:
$guid = [GUID]"3ab39606-c642-489b-84b6-58c038d3ef39"
Another curiosity. Although they have nothing to do with ImmutableIDs und local AD object GUIDs, there are some generic GUIDs in AzureAD also. Each object in the cloud (user accounts, groups, contacts, applications, roles, service principals….) has an ObjectID property, which is technically a GUID. But there is a difference in how this property is received if you ask for it in different PowerShell modules. If you use the AzureAD PowerShell module, the GUID is returned as a string:
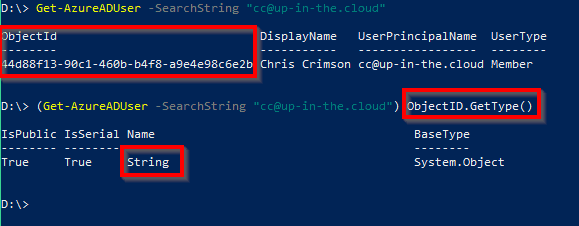
If you use the old MSOL module, it is returned as a pure GUID:
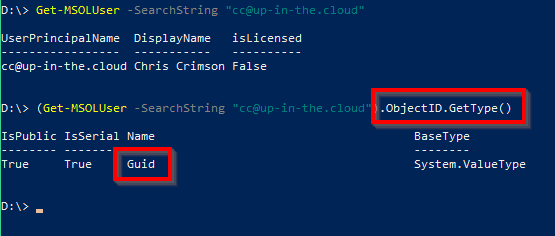