If you are leveraging an AADC server to sync your accounts to Azure AD, one of the most important technical properties is the source anchor. This is the value that matches the on-prem account with the cloud account. The nature of this match was discussed here in detail in another blog post: The ImmutableID Match in AADC environments.
So you store source anchor values in attributes like ImmutableID, objectGUID, or particularly in mS-DS-ConsistencyGUID. Normally source anchor values are GUIDs. But once they are saved as Base64 encoded values, then again as Hex Strings, or you have to deal with them as native GUIDs. If you deal with migrations from one tenant to another, or from one on-prem AD to another, you will always need to convert all these types of GUID representations – in all directions. The solution is my conversion carousel. Move the mouse over the image! 🙂
Why do I need ‘Hex String’ ?
Hex String is a string notation of a binary value – in hexadecimal characters, 2 chars per byte. In our case separated by blanks. So this is an example of an hex string:
74 E5 D2 BC 58 8D 36 41 A9 DF 8A 19 B1 45 DB 79
You may need this whenever you read or copy a value from the (binary) attribute ‘mS-DS-ConsistencyGUID‘. In the attribute editor in the ‘AD Users and Computers’ tool, the value is presented as a blank-separated hex string:
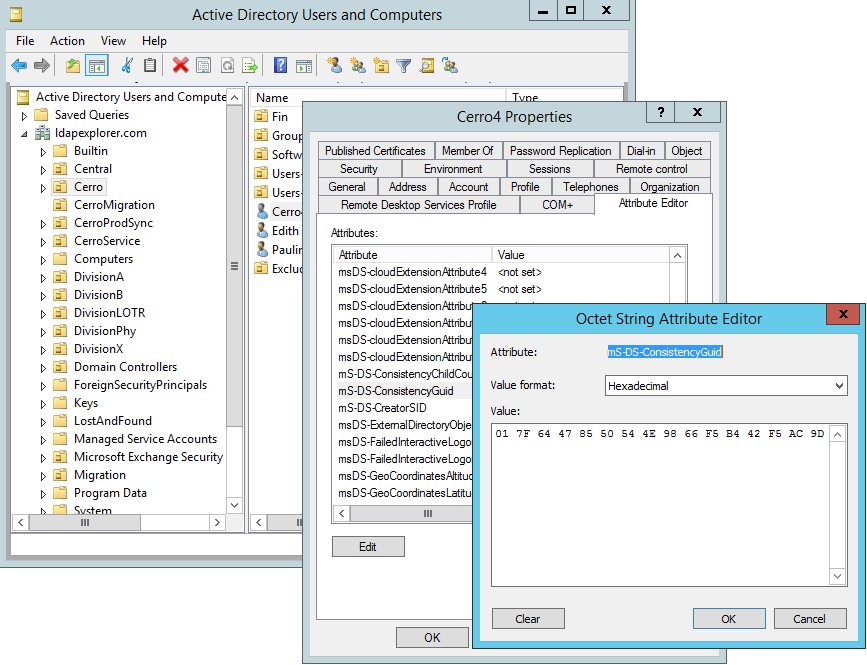
Or you need to search for an on-prem object with a certain GUID or mS-DS-ConsistencyGUID. In the LDAP filter, you have to use an Hex String with a backslash before each byte:
(objectGUID=\74\E5\D2\BC\58\8D\36\41\A9\DF\8A\19\B1\45\DB\79)
(mS-DS-ConsistencyGUID=\74\E5\D2\BC\58\8D\36\41\A9\DF\8A\19\B1\45\DB\79)
GUID -> Base64
$guid = "bcd2e574-8d58-4136-a9df-8a19b145db79" # <- set your own GUID string here
$base64 = [system.convert]::ToBase64String(([GUID]$guid).ToByteArray())
$base64
For example, if you have a GUID value from an on-premise AD account and want to know how the ImmutableID value of the synced cloud user looks like….
Base64 -> GUID
$base64 = "dOXSvFiNNkGp34oZsUXbeQ==" # <- set your own Base64 string here
$guid = [GUID]([system.convert]::FromBase64String($base64))
$guid
You’ve got an ImmutableID value, and you need the GUID? This is for you then. The result is a real .NET GUID type. If you need it as string, do this:
$guid.ToString()
Hex String -> Base64
$hexstring = "74 E5 D2 BC 58 8D 36 41 A9 DF 8A 19 B1 45 DB 79" # <- set your own Hex string here
$base64 = [system.convert]::ToBase64String([byte[]] (-split (($hexstring -replace " ", "") -replace '..', '0x$& ')))
$base64
You may need this whenever you copy a value from the (binary) attribute ‘mS-DS-ConsistencyGUID’ from the Admin GUI tools. And then you need a Base64 representation for setting/searching the cloud ImmutableID somewhere.
Base64 -> Hex String
$base64 = "dOXSvFiNNkGp34oZsUXbeQ==" # <- set your own Base64 stringhere
$hexstring = ([system.convert]::FromBase64String($base64) | % ToString X2) -join ' '
$hexstring
You will need this if you got an ImmutableID value from a cloud account (Base64), and you need the hex string to set the mS-DS-ConsistencyGUID value in the Attribute Editor of the ‘AD Users and Computers tool’.
Or you need to search for an on-prem object which is matched to the cloud account with a certain ImmutableID (Base64). For an appropriate LDAP filter, just replace the blanks with backslashes:
$base64 = "dOXSvFiNNkGp34oZsUXbeQ=="
$hexstring = ([system.convert]::FromBase64String($base64) | % ToString X2) -join ' '
$hexstring
$ldapFilter = "(mS-DS-ConsistencyGUID=\" + ($hexstring -replace " ", "\") + ")"
$ldapFilter
Import-Module ActiveDirectory
Get-ADUser -LdapFilter $ldapFilter
You can of course also search for accounts with a specific object GUID in this way. To do this, simply replace the mS-DS-ConsistencyGUID in the LDAP filter with ‘objectGUID’:
...
$ldapFilter = "(objectGUID=\" + ($hexstring -replace " ", "\") + ")"
...
GUID -> Hex String
$guid = "bcd2e574-8d58-4136-a9df-8a19b145db79" # <- set your own GUID string here
$hexstring = (([GUID]$guid).ToByteArray() | % ToString X2) -join ' '
$hexstring
You will need this if you got an object GUID, and you need the hex string to set the GUID as an mS-DS-ConsistencyGUID value in the Attribute Editor of the ‘AD Users and Computers tool’.
Or you need to search for an on-prem AD user with exactly this GUID in its attributes. For an appropriate LDAP filter, just replace the blanks with backslashes:
$guid = "bcd2e574-8d58-4136-a9df-8a19b145db79" # <- set your own GUID string here
$hexstring = (([GUID]$guid).ToByteArray() | % ToString X2) -join ' '
$hexstring
$ldapFilter = "(mS-DS-ConsistencyGUID=\" + ($hexstring -replace " ", "\") + ")"
$ldapFilter
Import-Module ActiveDirectory
Get-ADUser -LdapFilter $ldapFilter
Hex String -> GUID
$hexstring = "74 E5 D2 BC 58 8D 36 41 A9 DF 8A 19 B1 45 DB 79" # <- set your own Hex string here
$guid = [GUID]([byte[]] (-split (($hexstring -replace " ", "") -replace '..', '0x$& ')))
$guid
You may need this whenever you copy a value from the (binary) attribute ‘mS-DS-ConsistencyGUID’ from the Admin GUI tools. And then you want to know which GUID this is. The result is a real .NET GUID type. If you need it as string, do this:
$guid.ToString()
Binary -> Hex String
$binary = [byte[]](116,229,210,188,88,141,54,65,169,223,138,25,177,69,219,121) # <- set your own binary byte array here
$hexstring = ($binary | % ToString X2) -join ' '
$hexstring
If you get the attribute ‘mS-DS-ConsistencyGUID’ by script, it is pure binary (array of bytes). You may want to transfer this to some other form – this one-liner does the job.

Hex String -> Binary
$hexstring = "74 E5 D2 BC 58 8D 36 41 A9 DF 8A 19 B1 45 DB 79" # <- set your own Hex string here
$binary = [byte[]] (-split (($hexstring -replace " ", "") -replace '..', '0x$& '))
$binary
You need the binary form whenever you want to write the attribute ‘mS-DS-ConsistencyGUID’ by script to an on-prem user account. Beause in the ‘Set-ADUser’ cmdlet, you have to pass a binary value – a byte array:

The Flex Converter Script
When I deal with those GUID values and have to convert them often during a migration project, I often use this script below. I call it “Flex Converter”, because it takes any value (GUID string, HEX string, or Base64), detects what it is and converts it into the other data representations:
[void][Reflection.Assembly]::LoadWithPartialName('Microsoft.VisualBasic')
$title = 'GUID Converter'
$msg = 'Enter your GUID / Hex String / Base64:'
$text = [Microsoft.VisualBasic.Interaction]::InputBox($msg, $title)
if ($text.Contains('-')) {
$guid = $text
$base64 = [system.convert]::ToBase64String(([GUID]$guid).ToByteArray())
$hexstring = (([GUID]$guid).ToByteArray() | % ToString X2) -join ' '
} elseif ($text.Contains(' ')) {
$hexstring = $text
$guid = [GUID]([byte[]] (-split (($hexstring -replace ' ', '') -replace '..', '0x$& ')))
$base64 = [system.convert]::ToBase64String([byte[]] (-split (($hexstring -replace ' ', '') -replace '..', '0x$& ')))
} else {
$base64 = $text
$guid = [GUID]([system.convert]::FromBase64String($base64))
$hexstring = ([system.convert]::FromBase64String($base64) | % ToString X2) -join ' '
}
""
$guid.ToString()
$base64
$hexstring
5 thoughts on “The GUID Conversion Carousel”
Exactly what I was looking for! Helped me be able to look at my on-prem AD user objects and know what the Immutable ID should be in AAD.
Well done great article
Thanks,
It was very usefull for me. My needs was to extract objectGUID in HEX format with “\”.
This is pure gold!
Can’t believe this incredibly helpful and comprehensive post hasn’t attracted a single comment yet. Anyway thanks, it has helped me immensely during my current project setting up some automated local AD housekeeping.